By: AY1920S2-CS2103T-F09-4
Since: Jan 2020
Licence: MIT
- 1. Introduction
- 2. Setting up
- 3. Design
- 4. Implementation
- 4.1. View Switching for Navigation Commands
- 4.2. Specific Help Windows for different pages
- 4.3. Adding a trip in Home Page
- 4.4. Sorting entries in Journal Page
- 4.5. Adding activities in Itinerary Page
- 4.6. Finding activities in Itinerary Page
- 4.7. Deleting Trip in Home Page
- 4.8. Adding an activity in Itinerary Page
- 4.9. Finding activities in Itinerary Page
- 4.10. Refreshing trips in Home Page
- 4.11. Data Storage
- 4.12. Logging
- 4.13. Configuration
- 5. Documentation
- 6. Testing
- 7. Dev Ops
- Appendix A: Product Scope
- Appendix B: User Stories
- Appendix C: Use Cases
- Appendix D: Non Functional Requirements
- Appendix E: Glossary
- Appendix F: Product Survey
- Appendix G: Instructions for Manual Testing
1. Introduction
This document is a Developer Guide written for developers who wish to contribute to or extend our project. It is technical, and explains the inner workings of Volant and how the different components of our application work together.
Reading this Developer Guide
-
Important Notations
This icon denotes useful tips to note of during development. This icon denotes important details to take note of during development. -
Understanding Diagrams
The diagrams in this Developer Guide are colour coded according to the different components.
2. Setting up
Refer to the guide here.
3. Design
3.1. Architecture
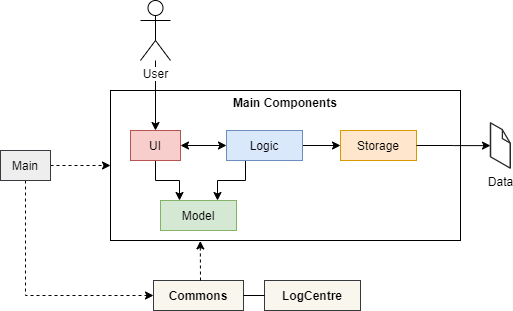
The Architecture Diagram given above explains the high-level design of the App. Given below is a quick overview of each component.
-
At app launch: Initializes the components in the correct sequence, and connects them up with each other.
-
At shut down: Shuts down the components and invokes cleanup method where necessary.
-
Commons
represents a collection of classes used by multiple other components. The following class plays an important role at the architecture level: -
LogsCenter
is used by many classes to write log messages to the App’s log file,volant.log
.
The rest of the App consists of four main components.
Each of the four components
-
Defines its API in an
interface
with the same name as the Component. -
Exposes its functionality using a
{Component Name} Manager
class.
For example, the Logic
component (see the class diagram given below) defines its
API in the Logic.java
interface and exposes its functionality using the LogicManager.java
class.
3.1.1. Views in Volant
There are 4 types of pages/views in this App: HOME
, TRIP
, ITINERARY
, JOURNAL
.
Each view has their own {Component Name} Manager
classes for both Logic
and Model
.
These individual {Component Name} Manager
classes for Logic
and Model
provide a context for commands to be executed in,
e.g. when the user is on the HOME
page, the add
command modifies the trip list, when the user
is on the ITINERARY
page, the add
command modifies the itinerary.
For example, the JOURNAL
view has its own JournalLogicManager
and JournalModelManager
to provide the context
for command execution on the JOURNAL
page.
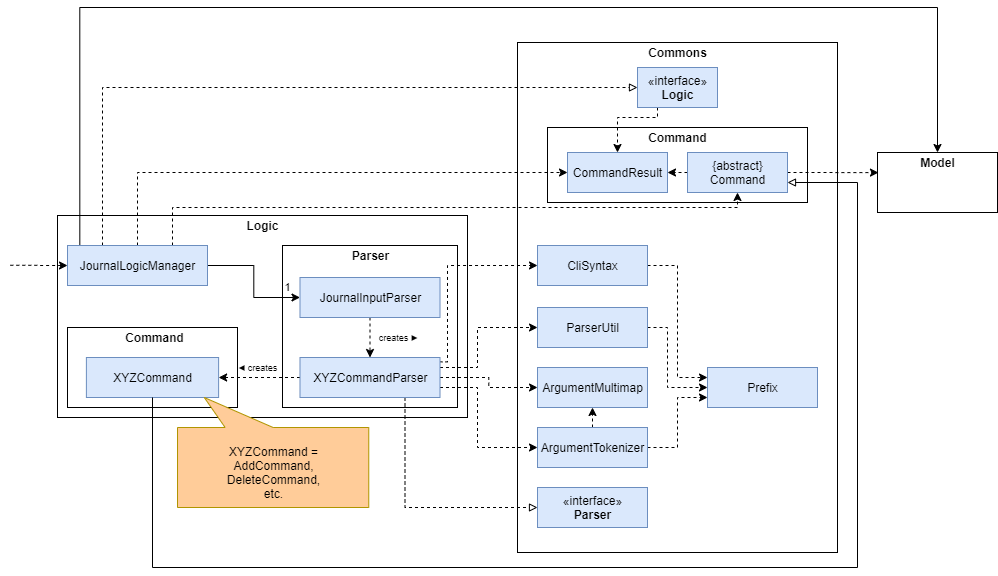
How the architecture components interact with each other
The Sequence Diagram below shows how the components interact with each other for the scenario where the user issues
the command back
when in a TRIP
view. View switching is executed by the Ui
component.
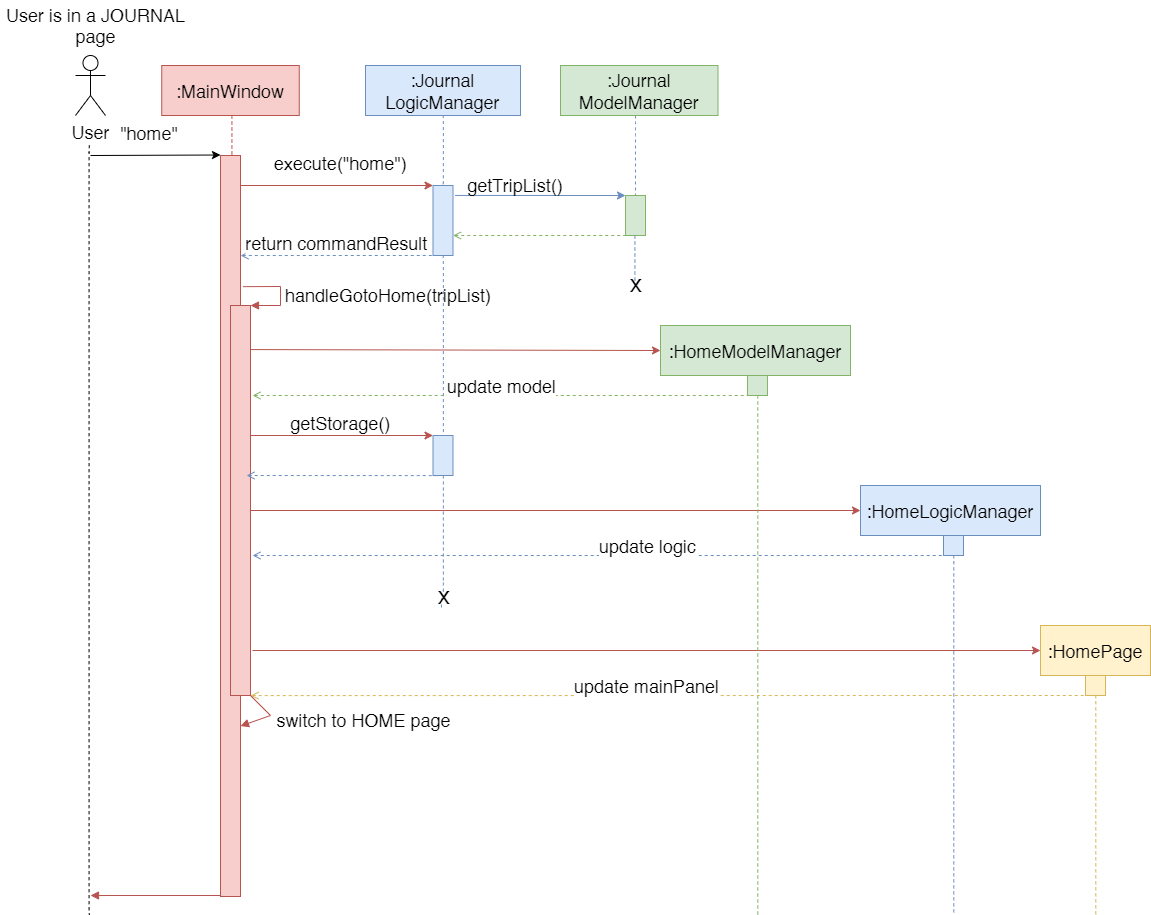
home
command in the JOURNAL
page.The Sequence Diagram below illustrates how the components interact with each other for the scenario where the user
issues the command goto 1
when in a HOME
page view.
View switching is executed by the Ui
component.
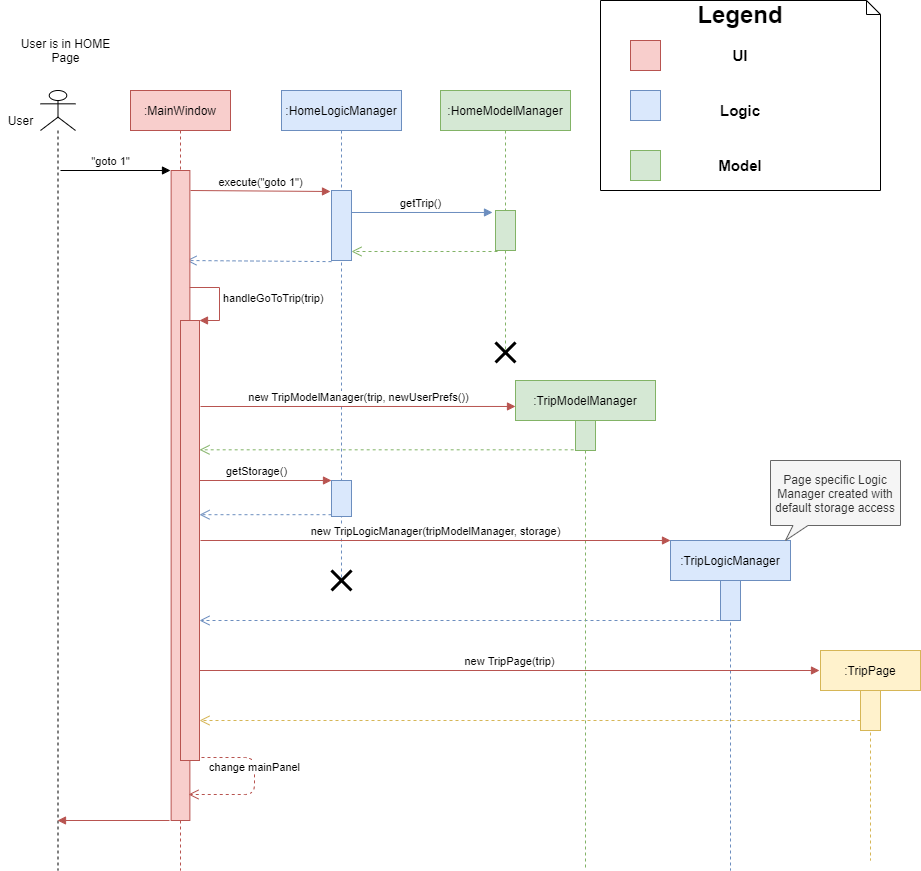
goto 1
command in the HOME
page view.The sections below give more details about each component.
3.2. UI component
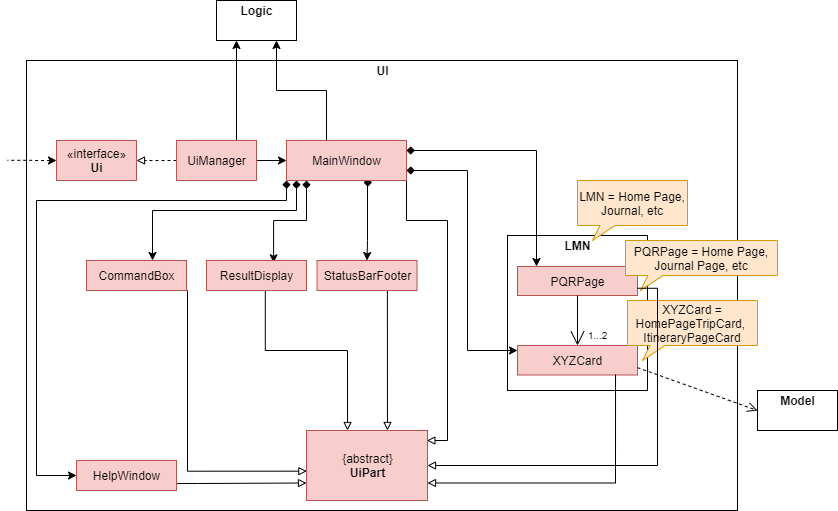
TRIP
pageAPI : Ui.java
The UI consists of a MainWindow
that is made up of parts e.g.CommandBox
, ResultDisplay
, StatusBarFooter
, mainPanel
etc. All these, including the MainWindow
, inherit from the abstract UiPart
class.
The UI
component uses JavaFx UI framework. The layout of these UI parts are defined in matching .fxml
files that are in the src/main/resources/view
folder. For example, the layout of the MainWindow
is specified in MainWindow.fxml
The UI
component,
-
Executes user commands using the
Logic
component. -
Listens for changes to
Model
data so that the UI can be updated with the modified data.
3.2.1. View Switching
As there are four different views in Volant. Upon startup of the App, the mainPanel
is set to HomePage
.
There are certain commands will cause the MainWindow
display to switch views.
For example, goto
, back
and home
navigation commands.
When a view is switched, MainWindow
will do the following:
For example, if a user is switching from a TRIP
view to the HOME
view through the command, back
.
-
Reassign the
Logic
component fromTripPageLogicManager
to a newHomePageLogicManager
. -
Reassign the
Model
component fromTripPageModelManager
to a newHomePageModelManager
. -
Reassign the value of
StackPane
mainPanel
fromTripPage
toHomePage
.
More details on the implementation of view switching can be found in Section 4.1, “View Switching for Navigation Commands”.
3.3. Logic component
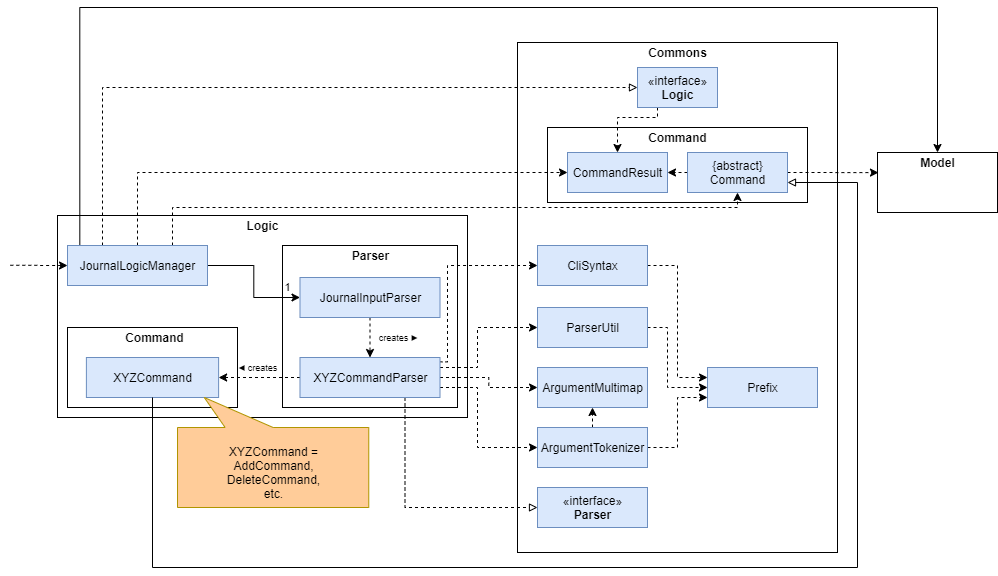
API :
Logic.java
-
Logic
uses theJournalInputParser
class of the current feature to parse the user command. -
This results in a
Command
object which is executed by theJournalLogicManager
of the current feature. -
The command execution can affect the
Model
of the current feature (e.g. adding a trip). -
The result of the command execution is encapsulated as a
CommandResult
object which is passed back to theUi
. -
In addition, the
CommandResult
object can also instruct theUi
to perform certain actions, such as displaying help to the user.
Given below is the Sequence Diagram for interactions within the Logic
component of the Itinerary feature for the execute("delete 1")
API call.
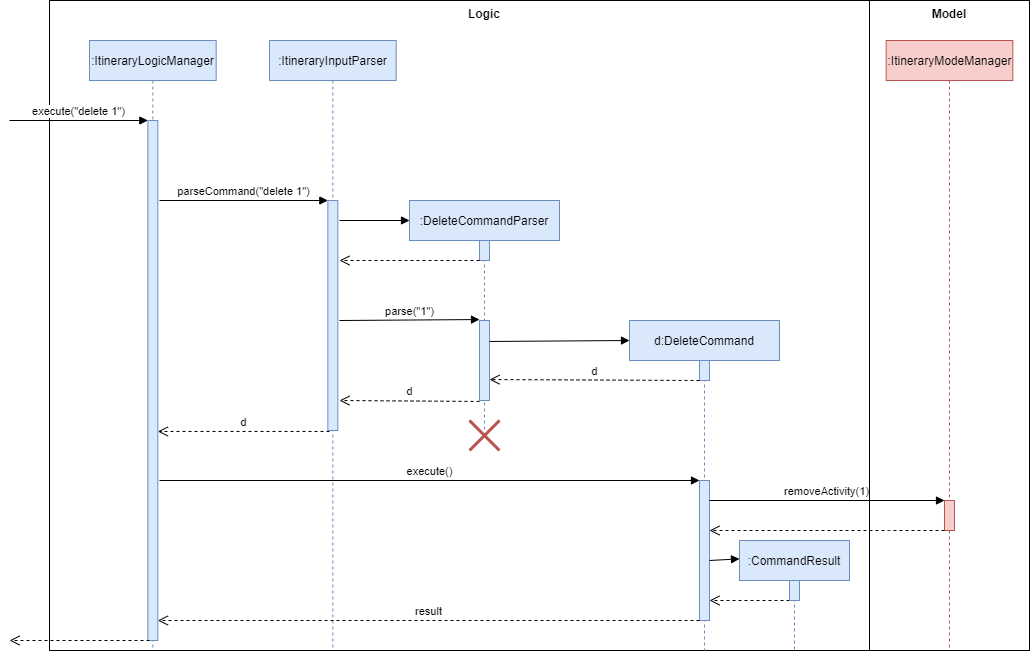
delete 1
Command3.4. Model component
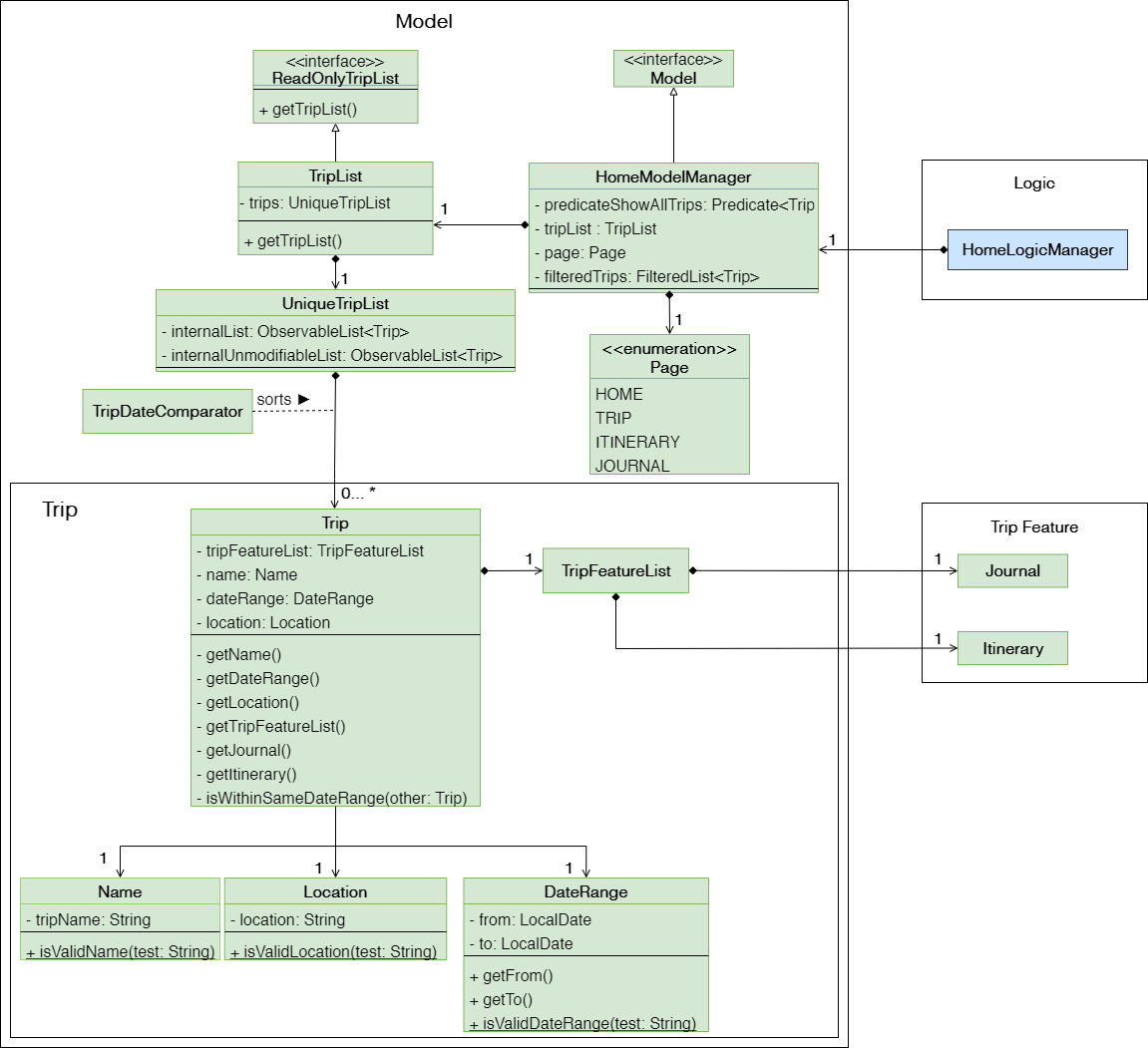
HOME
page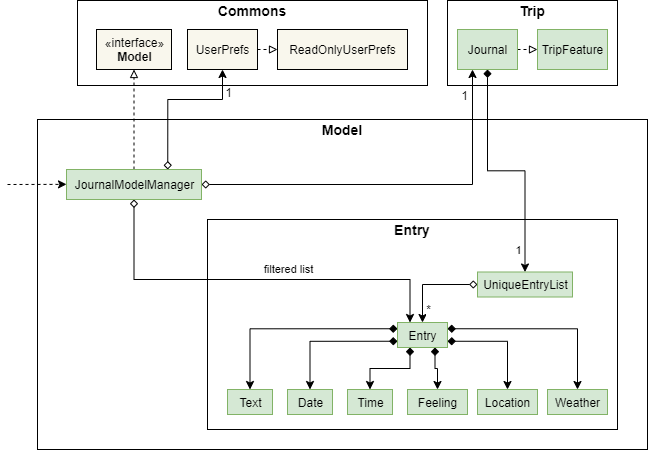
API : Model.java
The Model
for JOURNAL
,
-
stores a
UserPref
object that represents the user’s preferences. -
stores an
Entry
's data. -
exposes an unmodifiable
ObservableList<Entry>
that can be 'observed' e.g. the UI can be bound to this list so that the UI automatically updates when the data in the list change. -
does not depend on any of the other three components.
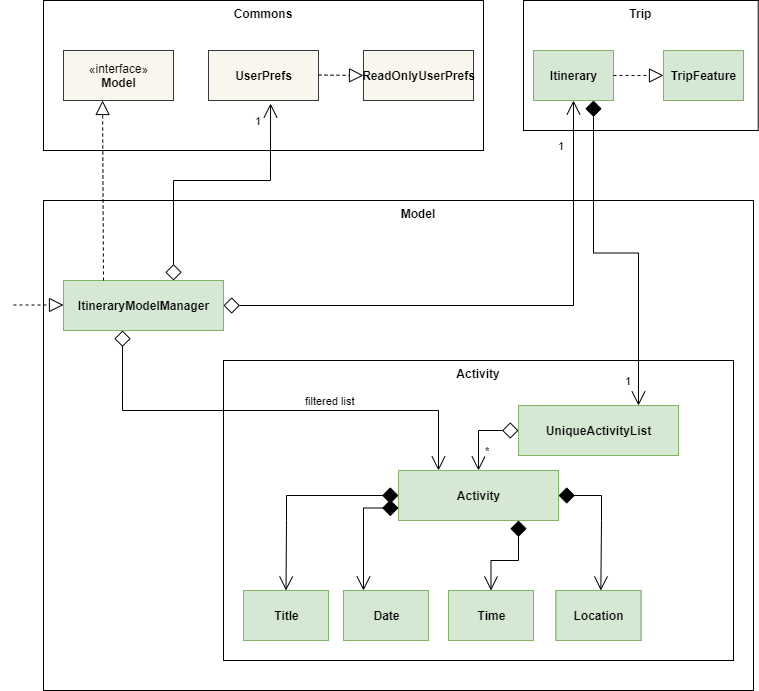
The Model
for ITINERARY
,
API : Model.java
-
stores a
UserPref
object that represents the user’s preferences. -
stores an
Activity
's data. -
exposes an unmodifiable
ObservableList<Activity>
that can be 'observed' e.g. the UI can be bound to this list so that the UI automatically updates when the data in the list change. -
does not depend on any of the other three components.
3.5. Storage component
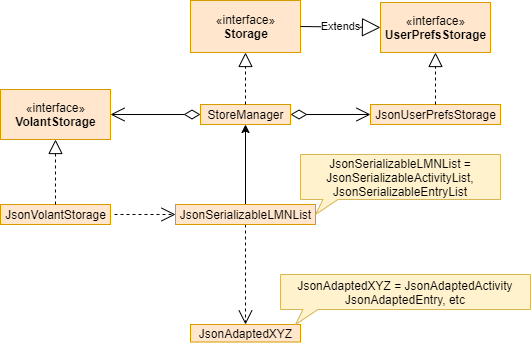
API : Storage.java
The Storage
component,
-
can save
UserPref
objects in json format and read it back. -
can save the
LMNList
data in json format and read it back.
3.6. Common classes
Classes used by multiple components are in the seedu.volant.commons
package.
4. Implementation
This section describes some noteworthy details on how certain features are implemented.
4.1. View Switching for Navigation Commands
The entire process of view switching is executed in the MainWindow.java
class.
List of Navigation Commands
|
4.1.1. Implementation
Each CommandResult
from an execution in the MainWindow
class stores data on
if a command is a navigation command or not, and which navigation command it is. If the command is a navigation command, the MainWindow
will execute the
appropriate functions to facilitate view switching.
In the view switching process, the MainWindow
will do the following:
-
Switch the logic component of the program to the logic component of the next page
-
Switch the model component of the program to the model component of the next page
-
Switch the current storage of the program to the storage component of the next page
-
Load the GUI components for the next page
For example, for the back
command, the MainWindow
:
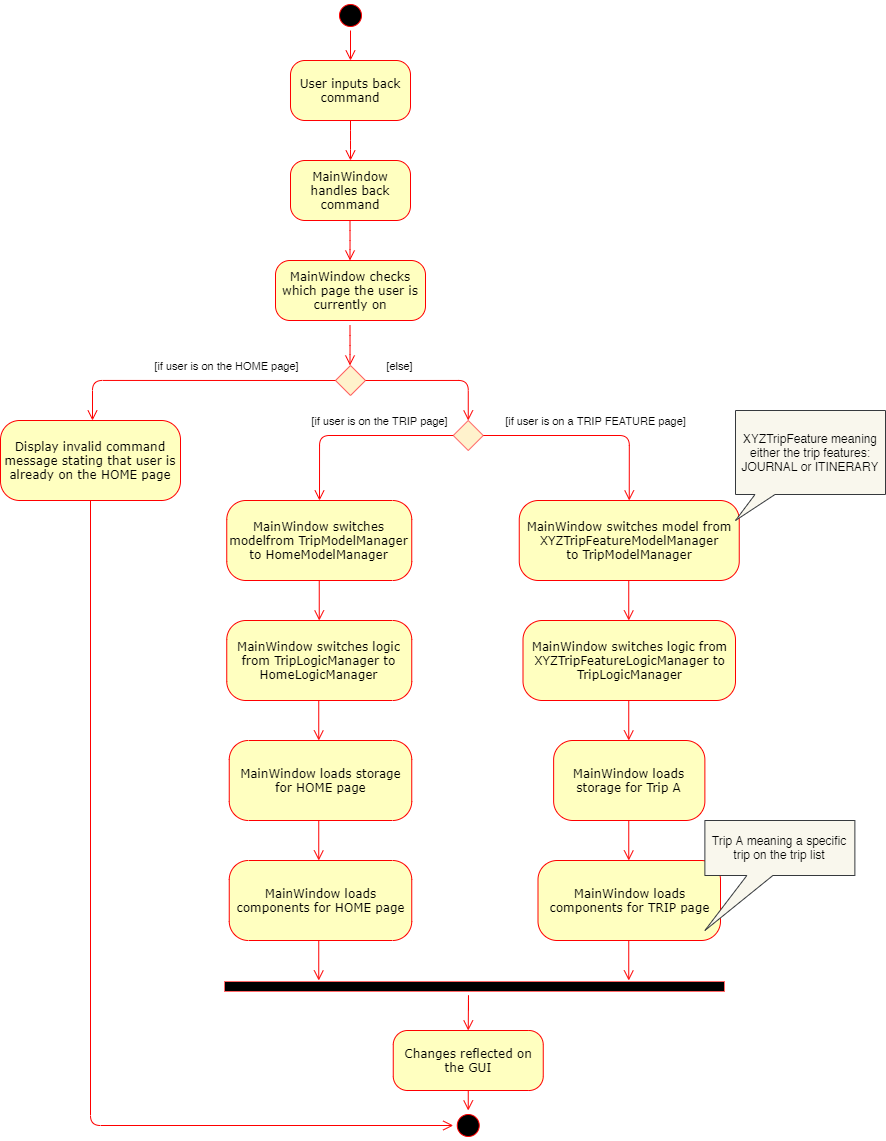
MainWindow
for back
command4.1.2. Design Considerations
Pros:
-
As each page has its own components, the back end of the program is neater and better abstracted.
-
Specialised logic components for each page allow for the same keywords to be used (e.g
add
,delete
) on certain pages.
Cons:
-
There are many steps to switching views
-
If there are more pages, there will be many logic and model components to keep track of.
4.1.3. Special Cases
-
When
back
command is used in the [root-page], this would not have any effect on the current GUI being displayed. -
When the
home
command is used in the [root-page], this would not have any effect on the current GUI being displayed as the user is already on the HOME page.
4.2. Specific Help Windows for different pages
4.2.1. Implementation
Each page in Volant has its own {Page}HelpWindow
class.
Upon switching views, the MainWindow
will create a new {Page}HelpWindow
containing
details of the commands that can be used on that page.
4.2.2. Design Considerations
Aspect: Syntax of the find command
-
Alternative 1 (current choice): Help Window specifically details the commands that can be used on the page that the user is currently on.
-
Pros: Very specific and allows added convenience for users when they want to use commands with many fields that they cannot remember without having to refer to the User Guide.
-
Cons: Every single page has to have its own class file for the Help Window, if there are many pages, there will be many files to keep track of.
-
-
Alternative 2: Use a general Help Window that lists every single command for every page in Volant
-
Pros: Only one Help Window class file needed and it is easy to implement.
-
Cons: If all the commands are detailed on a single long Help Window, the user will have to inconveniently scroll through the window to reach the section that details commands for the page that they are on.
-
4.3. Adding a trip in Home Page
4.3.1. Implementation
Adding a trip
The add trip feature allows user to add a trip to Volant. This feature is facilitated by HomeInputParser
, AddCommandParser
, and AddCommand
.
Given below is an example usage scenario and how the trip add mechanism behaves at each step:
-
The user executes the add command in
HOME
page and provides the required metadata in the correct format to be added. -
HomeInputParser
parses the input by the user to check if the input provided contains a valid command keyword. -
AddCommandParser
parses the remaining input by the user for metadata denoted with prefixes. -
AddCommandParser
checks if the metadata input by the user is in the desired format. -
AddCommandParser
creates a newAddCommand
based on the metadata provided. -
HomeLogicManager
executes theAddCommand
. -
HomeModelManager
checks if the newTRIP
has any logical conflicts with existingTRIP
(s) -
HomeModelManager
adds the module to theUniqueTripList
. -
A new folder with the
TRIP
name is created. -
HomeLogicManager
updates storage with updatedUniqueTripList
. -
MainWindow
receivesCommandResult
fromHomeLogicManager
containing success message to be displayed to user. -
Trips displayed in
MainWindow
are updated andMainWindow
displays success message.
The following activity diagram summarises what happens when a user executes a add trip command:
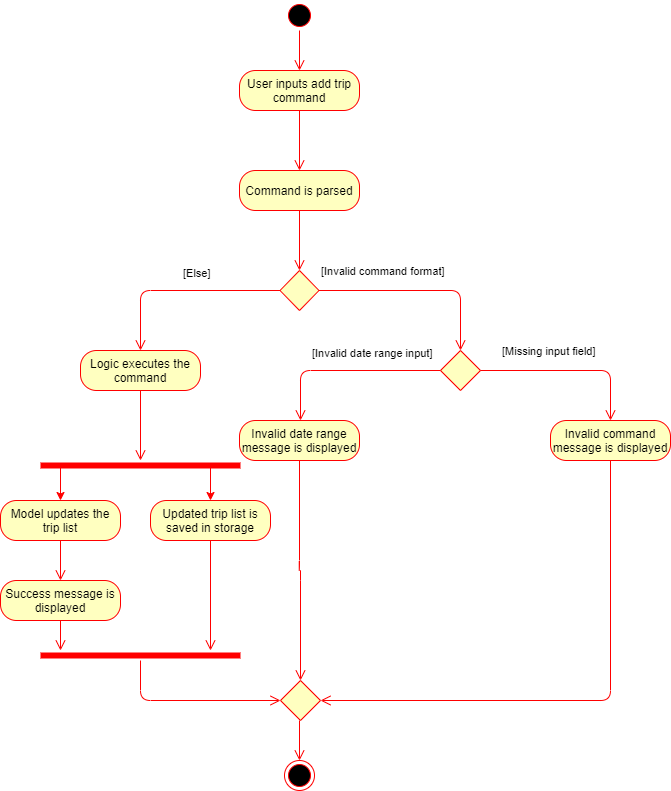
add
Command4.3.2. Design Considerations
Aspect: How data is stored
-
Alternative 1 (current choice): Saves the trip details in main volant json folder and create a folder corresponding to
TRIP
name.-
Pros: Easy to implement.
-
Cons: Less organised as all trip details are stored in one location. Any error in the way one trip is stored will cause all trips not to be loaded.
-
-
Alternative 2: Create a individual json file for each trip details.
-
Pros: An error in the file of one trip will not affect the loading of other trips.
-
Cons: Harder to implement retrieval.
-
4.4. Sorting entries in Journal Page
4.4.1. Implementation
Sorting journal entries
The sort feature allows user to sort the entries in the JOURNAL
of a trip. This feature is facilitated by JournalInputParser
, SortCommandParser
, and SortCommand
.
Given below is an example usage scenario and how the journal sort mechanism behaves at each step:
-
The user executes the sort command in
JOURNAL
page and provides a field which they want the entries to be sorted by. -
JournalInputParser
parses the input by the user to check if the input provided contains a valid command keyword. -
SortCommandParser
parses the remaining input by the user for field. -
SortCommandParser
checks if the field input by the user is a valid field to sort by. -
SortCommandParser
creates a newSortCommand
based on the field provided. -
JournalLogicManager
executes theSortCommand
. -
JournalModelManager
sorts the entries according to the input field. -
JournalLogicManager
updates storage with updatedUniqueEntryList
. -
MainWindow
receivesCommandResult
fromHomeLogicManager
containing success message to be displayed to user. -
Jounral entries displayed in
MainWindow
are updated andMainWindow
displays success message.
The following activity diagram summarizes what happens when a user executes a sort journal command:

sort
Command4.5. Adding activities in Itinerary Page
4.5.1. Implementation
Adding an activity
The add activity feature allows user to add an activity to a current trip. This feature is faciliated by ItineraryInputParser
, AddCommandParser
, and AddCommand
.
Given below is an example usage scenario and how the activity add mechanism behaves at each step:
-
The user executes the add command in
ITINERARY
page and provides the required metadata in the correct format to be added. -
ItineraryInputParser
parses the input by the user to check if the input provided contains a valid command keyword. -
AddCommandParser
parses the remaining input by the user for metadata denoted with prefixes. -
AddCommandParser
checks if the metadata input by the user is in the desired format. -
AddCommandParser
creates a newAddCommand
based on the metadata provided. -
ItineraryLogicManager
executes theAddCommand
. -
ItineraryModelManager
checks if the newACTIVITY
has any logical conflicts with existingACTIVITY
(s) -
ItineraryModelManager
adds the activity to theUniqueActivityList
. -
The
itinerary.json
file in the current trip folder in storage is updated -
ItineraryLogicManager
updates storage with updatedUniqueActivityList
. -
MainWindow
receivesCommandResult
fromItineraryLogicManager
containing success message to be displayed to user. -
Activities displayed in
MainWindow
are updated andMainWindow
displays success message.
The following activity diagram summarizes what happens when a user executes a add activity command:
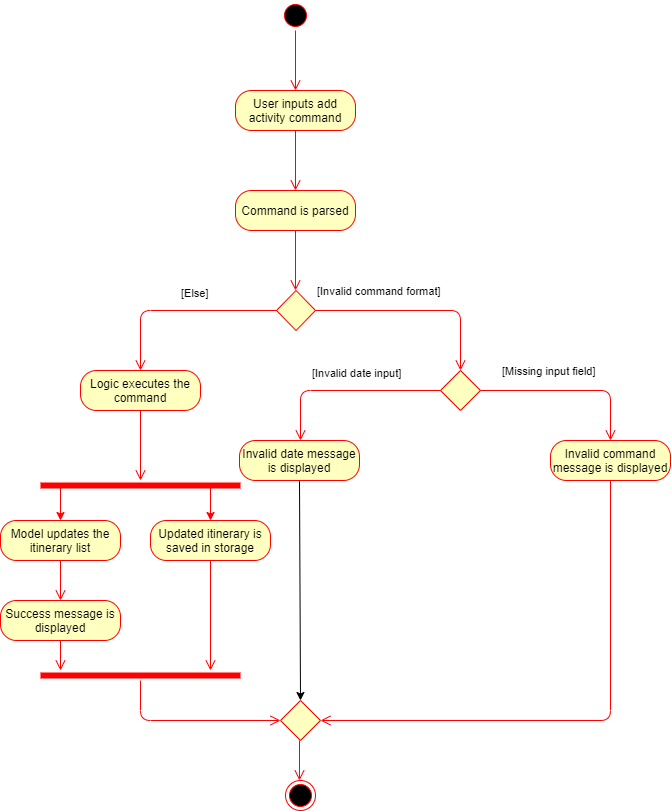
add
Command4.5.2. Design Considerations
Aspect: Prerequisite of the command
Since each trip is associated with one itinerary, when the user wants to use the add activity command, a trip must have already been created. This is why the order of the scence switching is important as it disallows user to create an activity that is not associated with any trip.
4.6. Finding activities in Itinerary Page
4.6.1. Implementation
Finding an activity
The find activity feature allows user to find one or several activities in the current trip with matching keywords provided. This feature is faciliated by ItineraryInputParser
, FindCommandParser
, and FindCommand
.
Given below is an example usage scenario and how the activity find mechanism behaves at each step:
-
The user executes the find command in
ITINERARY
page and provides one or several field(s) which they want to be displayed with the current keyword. -
ItineraryInputParser
parses the input by the user to check if the input provided contains a valid command keyword. -
FindCommandParser
parses the remaining input by the user for fields to be found. -
FindCommandParser
checks if the field input by the user is a valid field to find. -
FindCommandParser
creates a newFindCommand
based on the fields provided. -
ItineraryLogicManager
executes theFindCommand
. -
A new find predicate is created to check for matching keyword from the current list of activities.
-
ItineraryModelManager
checks the currentUniqueActivityList
to find activities with matching keywords provided based on the predicate created. -
ItineraryModelManager
updates the activities that matched in theFilteredActivityList
. -
MainWindow
receivesCommandResult
fromItineraryLogicManager
containing success message to be displayed to user. -
Jounral entries displayed in
MainWindow
are updated andMainWindow
displays success message.
The following activity diagram summarizes what happens when a user executes a find activity command:
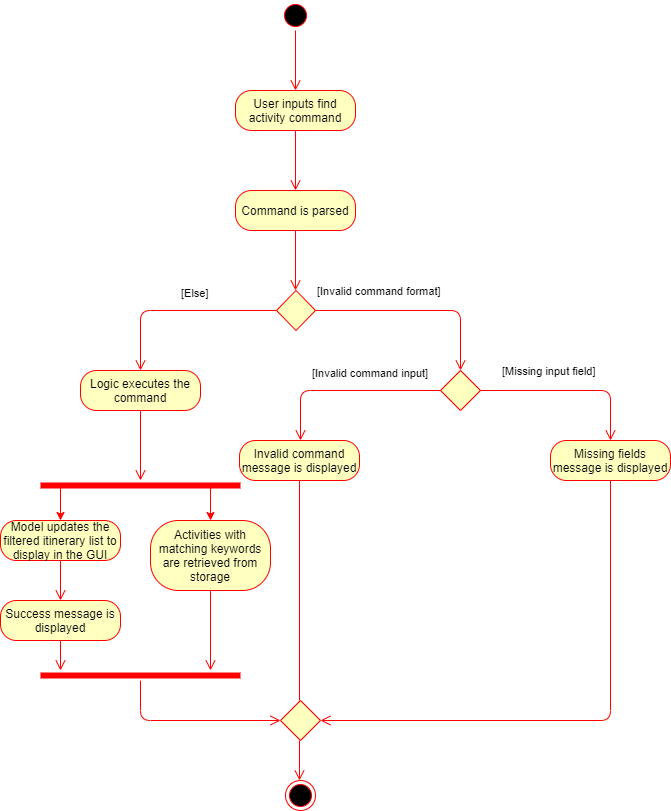
find
Command4.6.2. Design Considerations
Aspect: Syntax of the find command
-
Alternative 1 (current choice): Users are allowed to find according to specific fields. This is done by adding the prefixes(
t/
fortitle
,l/
forlocation
,d/
fordate
, andt/
fortime
) before the keyword to be found-
Pros: Allows users to be very specific in the find command. This means that users can find activities with the keywords in the specific field that they want. This implementation also allows users to append one find command with multiple fields to narrow down the search in less steps.
-
Cons: Cannot find the same keyword for multiple fields. The current implementation can also cause the CLI by the users to be longer as there is a need to specify the fields to find. Furthermore, this implementation is more troublesome to implement.
-
-
Alternative 2: Users can find with one or a few keywords without the need to specify the fields.
-
Pros: The commands would be a lot shorter and easy to use. This allows for cross-field search which is not possible with the current implementation. This implementation is also easier to implement.
-
Cons: This makes it difficult to narrow down the search if the users want to be specific about which field the keyword is in. The current implementation allows for greater freedom in choosing the fields to search for, something this implementation would not be able to accomplish. Furthermore, as
date
andtime
have different format fromlocation
andtitle
, the specified field would make it easier for users to search for activities with the samedate
ortime
.
-
The following activity diagram summarises what happens when a user executes a sort journal command:
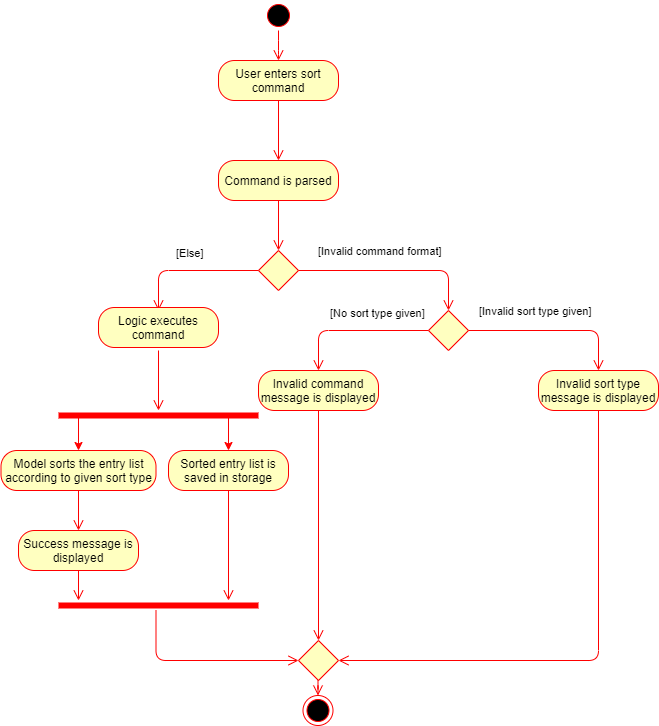
sort
Command4.7. Deleting Trip in Home Page
4.7.1. Implementation
The delete trip feature allows user to delete a trip in Volant. This feature is faciliated by HomeInputParser
, DeleteCommandParser
, and DeleteCommand
.
Given below is an example usage scenario and how the delete mechanism behaves at each step:
-
The user executes the delete command in
HOME
page and provides the desired index for deletion. -
HomeInputParser
parses the input by the user to check if the input provided contains a valid command keyword. -
DeleteCommandParser
parses the remaining input by the user for the index. -
DeleteCommandParser
checks if the index provided is valid; at most the number of trips in the list. -
DeleteCommandParser
creates a newDeleteCommand
with the index provided. -
HomeLogicManager
executes theDeleteCommand
. -
`The trip folder in the file path along with its contents are deleted during execution.
-
HomeModelManager
updates theUniqueTripList
. -
HomeLogicManager
updates storage with updatedUniqueTripList
. -
MainWindow
receivesCommandResult
fromHomeLogicManager
containing success message to be displayed to user. -
Trips displayed in
MainWindow
are updated andMainWindow
displays success message.
The following activity diagram summarises what happens when a user executes an add activity command:
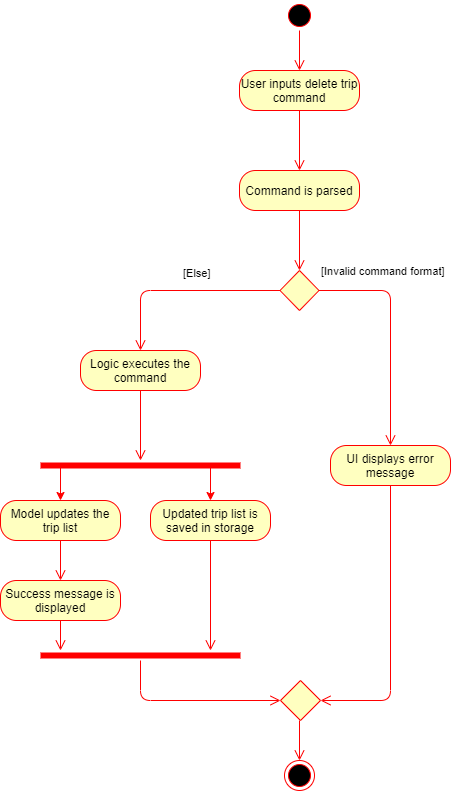
delete
Command4.7.2. Design Considerations
Aspect: How to delete the trip
Since each trip is a folder containing the data of the trip’s itinerary and journal. If the trip is deleted, the corresponding itinerary and journal json files should also be deleted. This prevents unnecessary files from being stored in the program.
4.8. Adding an activity in Itinerary Page
4.8.1. Implementation
The add activity feature allows user to add an activity to a current trip. This feature is faciliated by ItineraryInputParser
, AddCommandParser
, and AddCommand
.
Given below is an example usage scenario and how the activity add mechanism behaves at each step:
-
The user executes the add command in
ITINERARY
page and provides the required metadata in the correct format to be added. -
ItineraryInputParser
parses the input by the user to check if the input provided contains a valid command keyword. -
AddCommandParser
parses the remaining input by the user for metadata denoted with prefixes. -
AddCommandParser
checks if the metadata input by the user is in the desired format. -
AddCommandParser
creates a newAddCommand
based on the metadata provided. -
ItineraryLogicManager
executes theAddCommand
. -
ItineraryModelManager
checks if the newACTIVITY
has any logical conflicts with existingACTIVITY
(s) -
ItineraryModelManager
adds the activity to theUniqueActivityList
. -
The
itinerary.json
file in the current trip folder in storage is updated -
ItineraryLogicManager
updates storage with updatedUniqueActivityList
. -
MainWindow
receivesCommandResult
fromItineraryLogicManager
containing success message to be displayed to user. -
Activities displayed in
MainWindow
are updated andMainWindow
displays success message.
The following activity diagram summarises what happens when a user executes an add activity command:
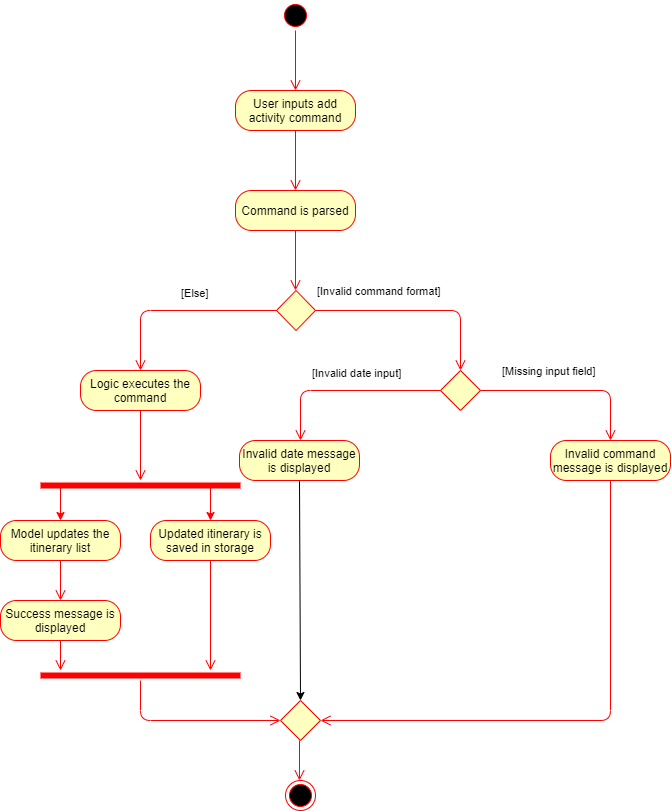
add
Command4.8.2. Design Considerations
Aspect: Prerequisite of the command
Since each trip is associated with one itinerary, when the user wants to use the add activity command, a trip must have already been created. This is why the order of the scence switching is important as it disallows user to create an activity that is not associated with any trip.
4.9. Finding activities in Itinerary Page
4.9.1. Implementation
The find activity feature allows user to find one or several activities in the current trip with matching keywords provided. This feature is faciliated by ItineraryInputParser
, FindCommandParser
, and FindCommand
.
Given below is an example usage scenario and how the activity find mechanism behaves at each step:
-
The user executes the find command in
ITINERARY
page and provides one or several field(s) which they want to be displayed with the current keyword. -
ItineraryInputParser
parses the input by the user to check if the input provided contains a valid command keyword. -
FindCommandParser
parses the remaining input by the user for fields to be found. -
FindCommandParser
checks if the field input by the user is a valid field to find. -
FindCommandParser
creates a newFindCommand
based on the fields provided. -
ItineraryLogicManager
executes theFindCommand
. -
A new find predicate is created to check for matching keyword from the current list of activities.
-
ItineraryModelManager
checks the currentUniqueActivityList
to find activities with matching keywords provided based on the predicate created. -
ItineraryModelManager
updates the activities that matched in theFilteredActivityList
. -
MainWindow
receivesCommandResult
fromItineraryLogicManager
containing success message to be displayed to user. -
Journal entries displayed in
MainWindow
are updated andMainWindow
displays success message.
The following activity diagram summarises what happens when a user executes a find activity command:
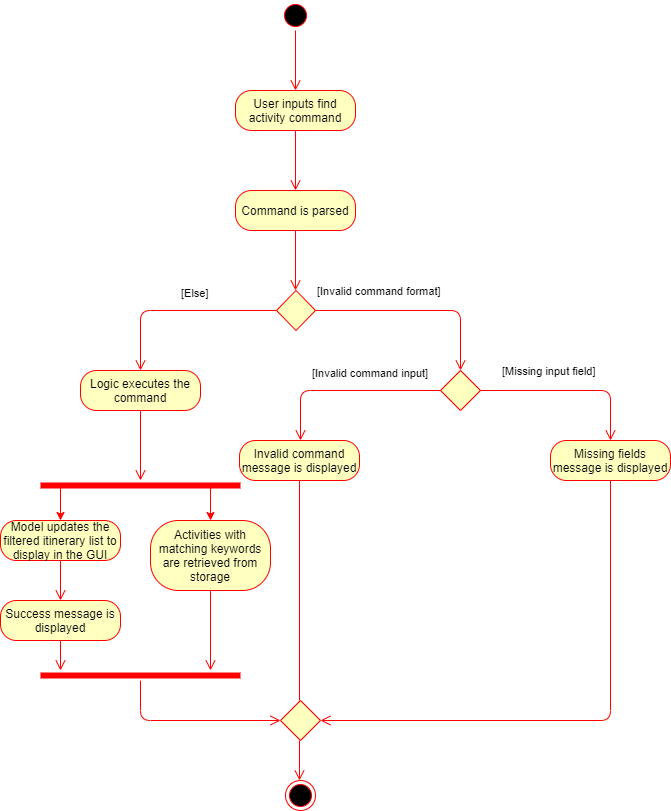
find
Command4.9.2. Design Considerations
Aspect: Syntax of the find command
-
Alternative 1 (current choice): Users are allowed to find according to specific fields. This is done by adding the prefixes(t/ for
title
, l/ forlocation
, d/ fordate
, and t/ fortime
) before the keyword to be found-
Pros: Allows users to be very specific in the find command. This means that users can find activities with the keywords in the specific field that they want. This implementation also allows users to append one find command with multiple fields to narrow down the search in less steps.
-
Cons: Cannot find the same keyword for multiple fields. The current implementation can also cause the CLI by the users to be longer as there is a need to specify the fields to find. Furthermore, this implementation is more troublesome to implement.
-
-
Alternative 2: Users can find with one or a few keywords without the need to specify the fields.
-
Pros: The commands would be a lot shorter and easy to use. This allows for cross-field search which is not possible with the current implementation. This implementation is also easier to implement.
-
Cons: This makes it difficult to narrow down the search if the users want to be specific about which field the keyword is in. The current implementation allows for greater freedom in choosing the fields to search for, something this implementation would not be able to accomplish. Furthermore, as
date
andtime
have different format fromlocation
andtitle
, the specified field would make it easier for users to search for activities with the samedate
ortime
.
-
4.10. Refreshing trips in Home Page
4.10.1. Implementation
The refresh feature allows user to repopulate the current page with the same data in the storage. This feature is faciliated by HomeInputParser
, RefreshCommandParser
, and RefreshCommand
.
Given below is an example usage scenario and how the refresh mechanism behaves at each step:
-
The user executes the rf command in
HOME
page. -
HomeInputParser
parses the input by the user to check if the input provided contains a valid command keyword. -
FindCommandParser
creates a newRefreshCommand
. -
HomeLogicManager
executes theRefreshCommand
. -
MainWindow
receivesCommandResult
and success message fromHomeLogicManager
. -
MainWindow
checks the current page user is on and repopulates display with list fromHomeModelManager
. -
MainWindow
displays success message.
The following activity diagram summarises what happens when a user executes a find activity command:
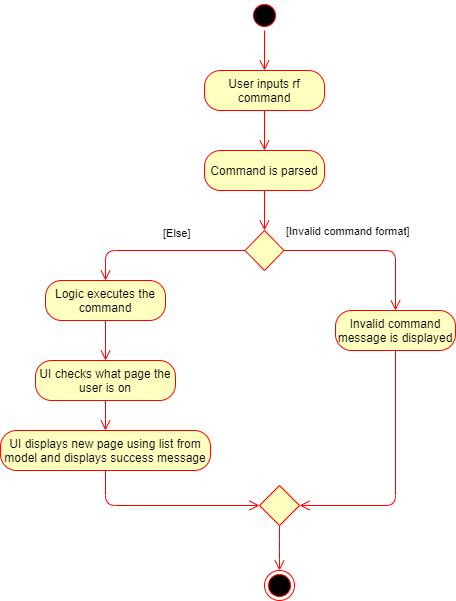
rf
Command4.10.2. Design Considerations
Aspect: How to get the list to repopulate the data with
After sorting or finding a list, the user needed a way to view the original data. The construction of each model includes a list that contains the relevant data in storage. Using that list, the view can be repopulated with the original data.
4.11. Data Storage
All data (trip details, journal entry details and itinerary details) is saved in the main.data
directory.
4.11.1. Organisation
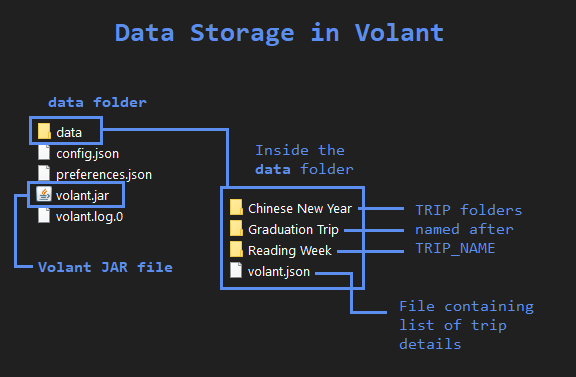
The data files are organised in a way that trip details are stored in volant.json
, while journal and itinerary details
are stored in a journal.json
file and a itinerary.json
file respectively. Both of these data files are organised
under a directory that represents the trip that the journal and itinerary are associated with.
The following explanation provides more details about these data files:
-
Within the
main.data
directory, thevolant.json
file contains a list of JSON objects, each representing a trip, with key-value pairs identifying the trip name, location and date range.Upon creation of a trip named "ABC", thevolant.json
file is updated with a new JSON object representing the trip. Themain.data.ABC
directory is also created. -
When an activity is added to the itinerary, a
itinerary.json
file is created in themain.data.ABC
directory, containing a list of JSON objects, each representing an activity, with key-value pairs identifying the activity title, location, date and time. -
When an entry is added to the journal, a
journal.json
file is created in themain.data.ABC
directory, containing a list of JSON objects, each representing a journal entry, with key-value pairs identifying the entry content, date, time, location, feeling and weather.
4.11.2. Design Considerations
Pros:
-
Each data file can be kept small in size.
-
This allows for faster retrieval of trip/journal/itinerary information from these data files.
Cons:
-
There is no single data file that provides an overview of all of a user’s data.
4.11.3. Data storage implementation: Adding a new trip/activity
Given below is an example usage scenario where a user adds a new trip:
-
The user executes the add command and provides the name, location and date range.
-
A new
Trip
object is created with the specified name, location and date range. -
In
HomeModelManager
, theTrip
object is added to theTripList
. -
A new folder is created in the
main.data
directory with the specified trip name. -
HomeLogicManager
accesses thevolant.json
file in themain.data
directory. -
Each
Trip
object in theTripList
is converted into aJsonAdaptedTrip
object. -
A new
JsonSerializableTripList
object is created with theJsonAdaptedTrip
objects. -
The new
JsonSerializableTripList
object is saved to thevolant.json
data file. -
The program log displays a success message.
The following activity diagram summarises what happens when a user adds a new trip:
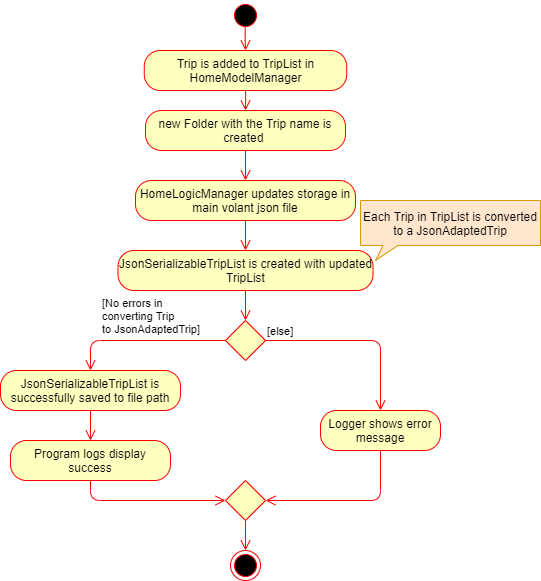
The process is similar for the scenario where the user adds a new activity to the itinerary, and the itinerary.json
data file is updated. The following activity diagram summarises this process:
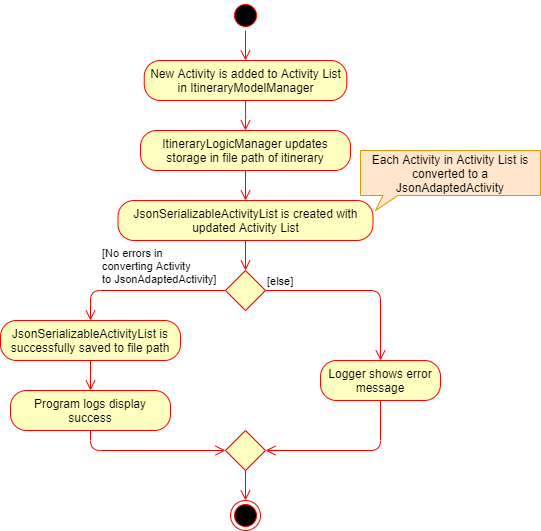
4.11.4. Data storage implementation: Editing a trip
Given below is an example usage scenario where a user edits a trip:
-
The user executes the edit command and provides the updated details.
-
HomeLogicManager
checks if the name of the trip was updated. If it was, a new directory will be created with the new trip name. Data files in the previous folder are moved into this new folder. Following that, the old folder is deleted. -
HomeLogicManager
accesses thevolant.json
file in themain.data
directory. -
Each
Trip
object in theTripList
is converted into aJsonAdaptedTrip
object. -
A new
JsonSerializableTripList
object is created with theJsonAdaptedTrip
objects. -
The new
JsonSerializableTripList
object is saved to thevolant.json
data file. -
The program log displays a success message.
The following activity diagram summarises what happens when a user edits a trip:
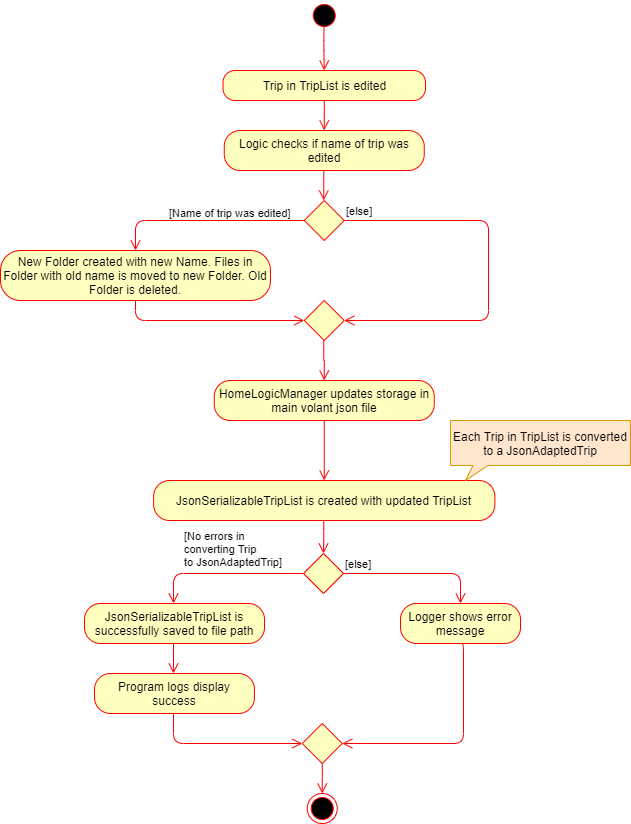
4.11.5. Data storage implementation: Deleting a journal entry/itinerary activity
Given below is an example usage scenario where a user deletes a journal entry:
-
The user executes the delete command.
-
In
JournalModelManager
, the identifiedEntry
is removed from theEntryList
. -
JournalLogicManager
accesses thejournal.json
file in the specific trip directory. -
Each remaining
Entry
object in theEntryList
is converted into aJsonAdaptedEntry
object. -
A new
JsonSerializableEntryList
object is created with theJsonAdaptedEntry
objects. -
The new
JsonSerializableEntryList
object is saved to thejournal.json
data file. -
The program log displays a success message.
This process is the same for scenarios where the user deletes an itinerary activity. The following activity diagram summarises what happens when a user deletes a journal entry or itinerary activity:
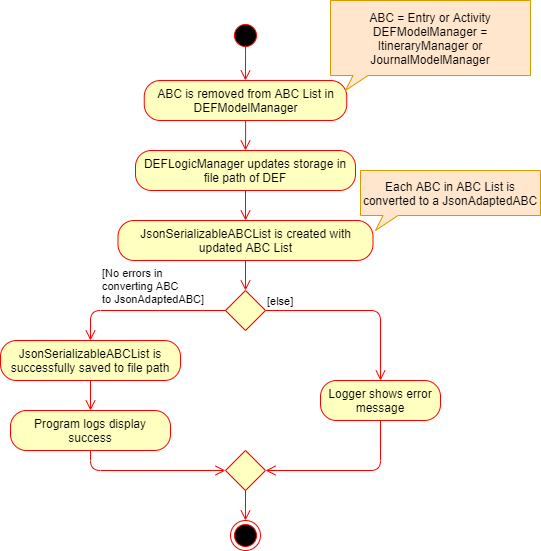
4.12. Logging
We are using java.util.logging
package for logging. The LogsCenter
class is used to manage the logging levels and logging destinations.
-
The logging level can be controlled using the
logLevel
setting in the configuration file (See Section 4.13, “Configuration”) -
The
Logger
for a class can be obtained usingLogsCenter.getLogger(Class)
which will log messages according to the specified logging level -
Currently log messages are output through:
Console
and to a.log
file.
Logging Levels
-
SEVERE
: Critical problem detected which may possibly cause the termination of the application -
WARNING
: Can continue, but with caution -
INFO
: Information showing the noteworthy actions by the App -
FINE
: Details that is not usually noteworthy but may be useful in debugging e.g. print the actual list instead of just its size
4.13. Configuration
Certain properties of the application can be controlled (e.g user prefs file location, logging level) through the configuration file (default: config.json
).
5. Documentation
Refer to the guide here.
6. Testing
Refer to the guide here.
7. Dev Ops
Refer to the guide here.
Appendix A: Product Scope
Target user profile:
-
is a solo traveller
-
prefers desktop apps over other types
-
can type fast
-
prefers typing over mouse input
-
is reasonably comfortable using CLI apps
Value proposition: convenient travel assistant to for solo travelers who are always on their feet.
Appendix B: User Stories
Priorities
-
High (must have) -
* * *
-
Medium (nice to have) -
* *
-
Low (not useful) -
*
Priority | As a … | I want to … | So that I can … |
---|---|---|---|
|
frequent traveler |
add a travel entry with weather, location and time metadata |
document my past travels |
|
traveler always on the go |
record short text entries recording my travels, similar to that of a Tweet on Twitter |
can keep track of my activities without excessive typing |
|
user |
add an activity into an itinerary list |
see my planned activities at a glance and better plan my trip |
|
user |
tag a location to each activity in the itinerary |
view where each activity is taking place |
|
user |
edit the entries in my itinerary list |
amend any mistakes when entering data, or change my travel plans |
|
user |
see a chronological timeline of the activities in the itinerary |
have a clear idea of my travel plans and schedule |
|
user |
view my itinerary and journal separately |
view them in a less cluttered manner |
|
frequent traveler |
see a record of all my past itineraries |
can review my past travels |
|
traveler always meeting new people overseas |
add a contact that I met during my trip with metadata including their name, age, phone number, location where I met them, and their country of origin |
can keep in contact with them |
|
user |
delete contacts |
get rid of contacts that I have not been in touch with for some time |
|
user |
view my itineraries, contact lists and journals specific to the trip they are relevant to |
effectively plan for multiple trips simultaneously |
|
user |
customize trip names |
distinguish between different trips that happen in the same location within similar date ranges |
|
frequent traveler |
to be able to see a timeline view of all my short journal entries and photos, including the location and time of the photos and entries, per trip |
relieve the memories of the trip in its original sequence |
|
food lover |
add a meal with description, photo, price and location |
keep track of what I ate |
|
user |
sort my travel entries by category depending on the ‘feeling’ tag of each travel entry |
see my best moments |
|
user |
record items in a packing list |
remind myself what I will need on a trip |
|
busy user |
list |
easily reference what I need for a trip at a glance |
|
fickle-minded user |
delete packing list entries |
remove items I feel I no longer need for the trip from the packing list |
{All user stories can be viewed in our issue tracker.}
Appendix C: Use Cases
(For all use cases below, the System is Volant (V)
and the Actor is the user
, unless specified otherwise)
UC01 - Adding New Trip
Precondition: Start from home page
Guarantee: A new Trip will be created
-
User enters details of trip to be added
-
V adds new trip to trip list
Use case ends
-
1a. V detects invalid characters in trip details
-
1a1. V outputs error informing user of invalid command
Use case ends
-
1b. V detects missing fields in trip details
-
1b1. V outputs error informing user of missing fields
Use case ends
-
1c. V detects that trip has a clashing date range with another trip on the trip list
-
1c1. V outputs error informing user of clashing date range between trip and another trip on the list
Use case ends
-
1d. V detects that trip has the same name as another trip on the trip list
-
1d1. V outputs error informing user that the trip already exists on the trip list
Use case ends
-
1e. V is unable to detect a valid command keyword
-
1e1. V outputs error informing user of invalid command
Use case ends
UC02 - Editing Existing Trip
Precondition: User is in the home page
Guarantee: Metadata of existing trip will be changed
MSS
-
User inputs command to edit an existing trip
-
V updates metadata of corresponding trip according to user input
-
Trip list is updated and the changes are reflected for user to see
Use case ends
Extensions
-
1a. V is unable to detect any specified fields to edit
-
1a1. V outputs error informing user that a field to edit must be specified
Use case ends
-
1b. V detects an invalid index i.e. index out of range or negative index
-
1b1. V outputs error informing user of invalid index specified
Use case ends
-
1c. V detects invalid characters for the fields
-
1c1. V outputs error informing user of invalid command
Use case ends
-
1d. V is unable to detect a valid command keyword
-
1d1. V outputs error informing user of invalid command
Use case ends
UC03 - Navigating to Trip Page
Precondition: User has added a trip
Guarantee: User will be moved to the Trip page
MSS
-
User changes directory to specific trip folder in trip list using index
-
V loads and displays trip page
Use case ends
Extensions
-
1a. V detects an invalid index i.e. index out of range or negative index
-
1a1. V outputs error informing user of invalid index specified
Use case ends
-
1b. V is unable to detect a valid command keyword
-
1b1. V outputs error informing user of invalid command
Use case ends
UC04 - Accessing Trip Feature Pages (Itinerary / Journal)
Precondition: User is in the home page
Guarantee: User will be moved to the desired page
MSS
-
User navigates to a specific trip (UC03)
-
User requests to navigate to the desired page in current trip
-
V loads and displays the desired page
Use case ends
Extensions
-
2a. V detects invalid field to navigate to the page
-
2a1. V outputs error informing user of invalid command
Use case ends
-
2b. V is unable to detect a valid command keyword
-
2b1. V outputs error informing user of invalid command
Use case ends
UC05 - Adding a travel Entry into Journal of a Trip
Precondition: User is already in the Journal page of Trip
Guarantee: A new travel entry will be added to the Travel Journal
MSS
-
User enters details of the travel entry
-
V confirms successful entry and displays new entry in the journal
Use case ends
Extensions
-
1a. V detects invalid characters in travel entry
-
1a1. V outputs error informing user of invalid command
Use case ends
-
1b. V detects missing fields in travel entry
-
1b1. V outputs error informing user of missing travel entry
Use case ends
-
1c. V is unable to detect a valid command keyword
-
1c1. V outputs error informing user of invalid command
Use case ends
-
1d. V detects that text field is greater 280 characters long
-
1d1. V outputs error informing user of character limit and how many characters over the limit their input was
Use case ends
UC06 - Adding Activities into Itinerary
Precondition: User is already in the Itinerary page of the Trip
Guarantee: A new activity will be added to the Itinerary
MSS
-
User enters details of activity
-
V confirms successful entry and displays updated itinerary
Use case ends
Extensions
-
1a. V detects invalid characters into activity entry
-
1a1. V outputs error informing user of invalid command
Use case ends
-
1b. V detects missing fields in activity entry
-
1b1. V outputs error informing user of missing activity entry
Use case ends
-
1c. V is unable to detect a valid command keyword
-
1c1. V outputs error informing user of invalid command
Use case ends
UC07 - Editing Existing Journal Entry
Precondition: User is on the Journal page and has added one or more entries already
Guarantee: Metadata of an existing entry will be changed
MSS
-
User inputs command to edit an entry
-
V updates metadata of corresponding entry according to user input
-
Entry list is updated and the changes are reflected for user to see
Use case ends
Extensions
-
1a. V is unable to detect any specified fields to edit
-
1a1. V outputs error informing user that a field to edit must be specified
Use case ends
-
1b. V detects incorrect date or time format
-
1b1. V outputs error informing user that the acceptable format for date and time input
Use case ends
-
1c. V detects an invalid index i.e. index out of range or negative index
-
1c1. V outputs error informing user of invalid index specified
Use case ends
-
1d. V detects invalid characters for the fields
-
1d1. V outputs error informing user of invalid command
Use case ends
-
1e. V is unable to detect a valid command keyword
-
1e1. V outputs error informing user of invalid command
Use case ends
UC08 - Editing Existing Activity
Precondition: User is on the Itinerary page and has added one or more activities already
Guarantee: Metadata of an existing activity will be changed
MSS
-
User inputs command to edit an activity.
-
V updates metadata of corresponding activity according to user input
-
Activity list is updated and the changes are reflected for user to see
Use case ends
Extensions
-
1a. V is unable to detect any specified fields to edit
-
1a1. V outputs error informing user that a field to edit must be specified
Use case ends
-
1b. V detects incorrect date or time format
-
1b1. V outputs error informing user that the acceptable format for date and time input
Use case ends
-
1c. V detects an invalid index i.e. index out of range or negative index
-
1c1. V outputs error informing user of invalid index specified
Use case ends
-
1d. V detects invalid characters for the fields
-
1d1. V outputs error informing user of invalid command
Use case ends
-
1e. V is unable to detect a valid command keyword
-
1e1. V outputs error informing user of invalid command
Use case ends
UC09 - Sorting Journal Entries
Precondition: User is on the Journal page and has added one or more entries already
Guarantee: Entry list will be sorted
MSS
-
User inputs sort command
-
V sorts the entry list according to user’s input
-
Entry list is updated and the sorted list is displayed to the user
Use case ends
Extensions
-
1a. V does not detect an argument for sort type in user’s input
-
1a1. V outputs error message informing user to include a sort type
Use case ends
-
1b. V detects invalid characters for the sort type input
-
1b1. V outputs error informing user of invalid sort type
Use case ends
-
1c. V is unable to detect a valid command keyword
-
1c1. V outputs error informing user of invalid command
Use case ends
UC10 - Finding Itinerary Activities
Precondition: User is on the Itinerary page and has added one or more activities already
Guarantee: Activity list containing matching search terms is displayed
MSS
-
User inputs find command
-
V checks the activity list for activities with metadata that matches the given search term(s)
-
V outputs an activity list containing all the activities matching the given search term(s)
Use case ends
Extensions
-
1a. V does not find any activities that match the given search term(s)
-
1a1. V outputs message informing user that no matching activities were found
Use case ends
-
1b. V detects invalid characters for the given search term(s)
-
1b1. V outputs error informing user of invalid command
Use case ends
-
1c. V is unable to detect a valid command keyword
-
1c1. V outputs error informing user of invalid command
Use case ends
Appendix D: Non Functional Requirements
-
Volant should work on any mainstream OS as long as it has Java
11
or above installed. -
Volant should work without any internet connection.
-
Volant should be able to hold up to 100 trips without noticing a increase in response time from the system for typical usage.
-
A user who is able to type above 40 words per minute (wpm) for regular English text (i.e. not code, not system admin commands) should be able to accomplish most of the tasks faster using commands than using the mouse.
-
Commands should be one-shot commands as opposed to multi-level commands.
-
A user should have minimum 100 Megabytes (MB) free disk space on their computer to store the program.
-
Input by the user should only be in English.
-
Volant source code should be covered by tests as much as possible.
-
Volant should work for a single user only.
Appendix E: Glossary
- Extensions
-
"add-on"s to the MSS that describe exceptional/alternative flow of events.
- Mainstream OS
-
Windows, Linux, Unix, OS-X
- MSS
-
Main Success Scenario
- Multi-level Commands
-
Commands that require multiple lines of user input for execution.
- Root Page
-
The first page the shows up when Volant is opened. By default, this is the
HOME
page. - One-shot Commands
-
Commands that are executed using only a single line of user input.
Appendix F: Product Survey
Volant
Author: Team Volant
- Pros
-
-
The product is effective in assisting solo travellers to plan and execute their trips.
-
GUI is very aesthetic looking, pleasing to the eyes.
-
The available commands are intuitive, and are easy to use and remember.
-
- Cons
-
-
The fremium model proposed can be a bit expensive.
-
A dark mode can be included. Some users prefer a GUI with dark mode.
-
More features can be integrated. These features can be included in version 2.0.
-
Appendix G: Instructions for Manual Testing
Given below are instructions to test the app manually.
These instructions only provide a starting point for testers to work on; testers are expected to do more exploratory testing. |
G.1. Launch and Shutdown
-
Initial launch
-
Download the jar file and copy into an empty folder.
-
Double-click the jar file.
Expected: Shows the GUI with a set of sample contacts. The window size may not be optimum.
-
-
Saving window preferences
-
Resize the window to an optimum size. Move the window to a different location. Close the window.
-
Re-launch the app by double-clicking the jar file.
Expected: The most recent window size and location is retained.
-
G.2. Adding
-
Adding a
TRIP
to an empty or populated trip list-
Prerequisites: NA
-
Test case:
add n/Bali 2020 l/Bali d/02-01-2020 to 02-05-2020
-
Expected:
TRIP
with corresponding details is added to trip list. -
Test case:
add n/Bali 2020 l/Bali d/2020-01-02 to 2020-05-02
-
Expected: No
TRIP
added. Error details shown in status message.
-
G.3. Deleting
-
Deleting a
TRIP
while allTRIPS
are listed-
Prerequisites: List all
TRIPS
using thelist
command. MultipleTRIPS
are present in the list. -
Test case:
delete 1
Expected: First contact is deleted from the list. Details of the deletedTRIP
are shown in the status message. Timestamp in the status bar is updated. -
Test case:
delete 0
Expected: NoTRIP
is deleted. Error details shown in the status message. Status bar remains the same. -
Other incorrect delete commands to try:
delete
,delete x
(where x is larger than the list size)
Expected: Similar to previous.
-
-
Deleting an
activity
in theITINERARY
-
Prerequisites: A
TRIP
has already been created. -
Test case:
delete 1
Expected: Firstactivity
is deleted from theactivity
list of theITINERARY
of the currentTRIP
. Currentactivity
list is updated. -
Test case:
delete 0
Expected: Noactivity
is deleted. Error details shown in the status message. -
Test case:
delete a
Expected: Noactivity
is deleted. Error details shown in the status message. -
Other incorrect delete commands to try:
delete
,delete x
(where x is larger than the itinerary size)
Expected: Similar to previous.
-
-
Deleting an
entry
in theJOURNAL
-
Prerequisites: A
TRIP
has already been created. -
Test case:
delete 1
Expected: Firstentry
is deleted from theJOURNAL
of the currentTRIP
. Currententry
list is updated. -
Test case:
delete 0
Expected: Noentry
is deleted. Error details shown in the status message -
Other incorrect delete commands to try:
delete
,delete x
(where x is larger than the journal size)
Expected: Similar to previous. Still
-